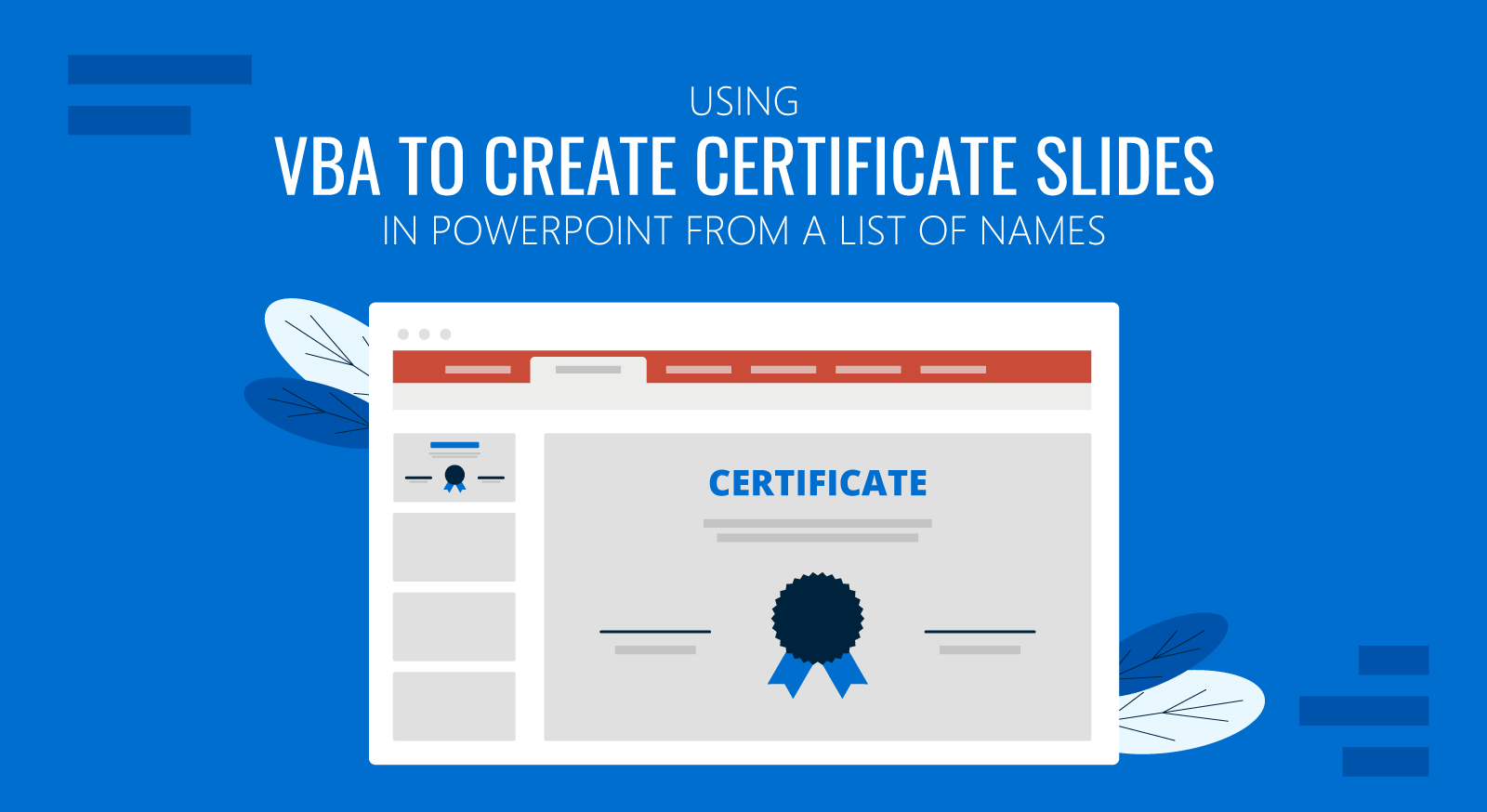
This article explores how you can use PowerPoint and Visual Basic for Applications (VBA) to automate the generation of certificates for groups of people.
Organizations frequently require personalized certificates for participants in events, courses, or training sessions. Microsoft PowerPoint is often used to generate those certificates, mainly because plenty of editable certificate templates are available that are very easy to edit. However, if we need to create tons of slides for participants, updating each participant’s name manually could be a tedious task, time-consuming and prone to errors. Here is where VBA comes into the rescue. And because SlideModel’s value proposition is to help their users to save time creating presentations, we present this idea of automating the process of creating certificates dynamically, which speeds up the design and ensures consistency in quality and format. We will also explain how you can make this VBA code using ChatGPT.
Basic Concepts of VBA and PowerPoint Integration
Visual Basic for Applications (VBA) is a powerful scripting language developed by Microsoft. It allows users to write functions, automate tasks, and manage data within the Office suite of products (not only in PowerPoint but also in Excel and Word, for example).
In Microsoft PowerPoint, VBA can be used to manipulate slide elements dynamically, creating opportunities for personalized content generation on a large scale and automating processes.
What do we need to generate certificates in bulk using PowerPoint?
Here are the key components required in our approach to generate certificates in bulk.
- A certificate presentation template. You can choose one of our certificate templates from the premium catalog of PowerPoint templates (or download this free Certificate PowerPoint template instead)
- The list of the participant’s names is saved in a text file
- A Macro-enabled presentation with the VBA code will load the list of names and generate a new slide for each participant’s name.
Once we understand the key elements of this approach, let’s see step-by-step the recipe for generating multiple certificate slides in bulk with the predefined list of names.
Step-by-Step How to Generate Certificate Slides in PowerPoint in Bulk using VBA
For the purpose of this example, we will use the scholarship certificate PPT template, that you can download from our catalog. You’d need to be an active users to download it, however if you want to try it on your own, you can download this free certificate PPT template (for PowerPoint & Google Slides) instead, and try it in your own.
1. Setting Up the Environment
To begin automating tasks with VBA in PowerPoint, you first need to enable the Developer tab:
- Open PowerPoint and click on File > Options.
- In the PowerPoint Options dialog box, select Customize Ribbon.
- Check the Developer checkbox and click OK.
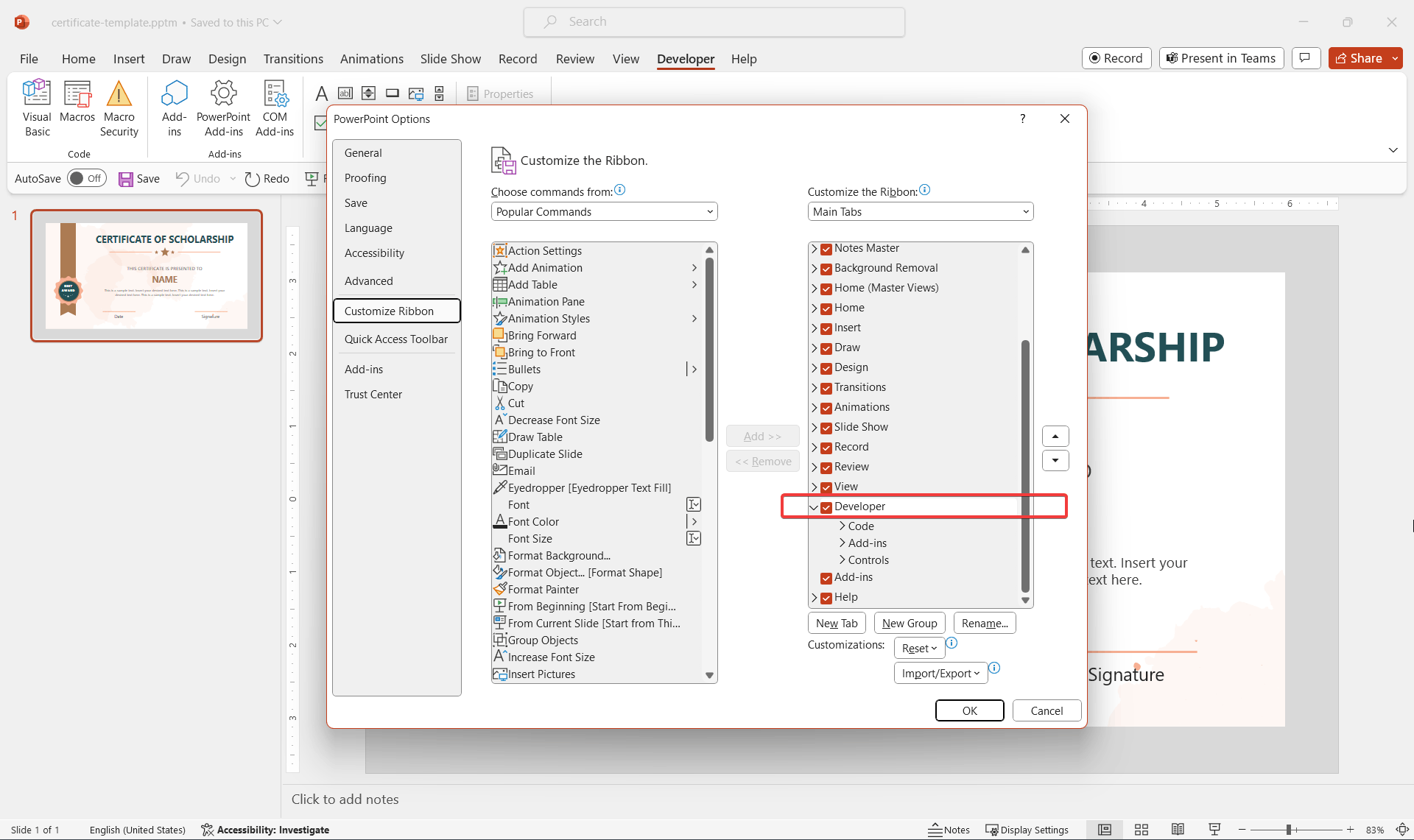
To access the VBA editor, go to the Developer tab and click on Visual Basic.
2. Creating the VBA Script
This step involves creating the VBA macro to generate certificates from data. In this step, we will create a script to generate multiple certificates.
Don’t you know how to code in VBA? Don’t worry, you can learn VBA (which is pretty straightforward if you have programming skills), and generate VBA code by leveraging LLMs (e.g. ChatGPT), or just download the code we will provide to you here (the easiest way).
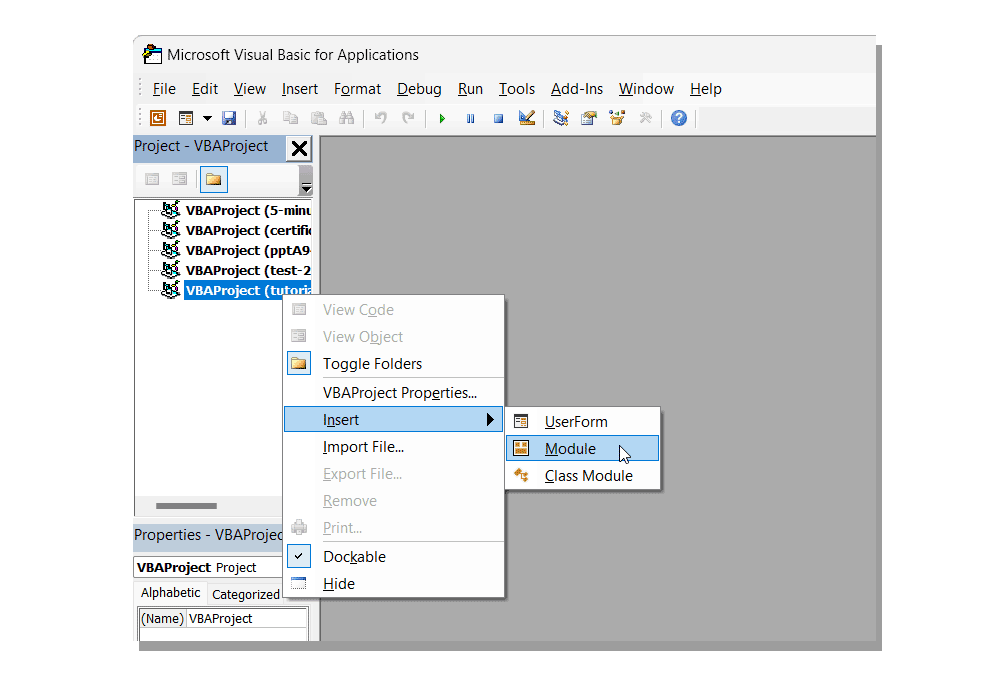
Then, it’s time to add the code to your presentation:
- Open the VBA Editor and insert a new Module.
- Paste the following code into the module, which automates reading names from a text file and populating a slide for each name. Alternatively, you can download the code from here.
Sub GenerateCertificates()
Dim fso As Object, ts As Object, textLine As String
Dim slideTemplate As Slide
Dim shapeName As String: shapeName = "FullName"
Dim fileDialog As fileDialog
Dim selectedFile As String
' Create a FileDialog object as a File Picker dialog box
Set fileDialog = Application.fileDialog(msoFileDialogFilePicker)
With fileDialog
.Title = "Select a Text File"
.Filters.Add "Text Files", "*.txt"
.AllowMultiSelect = False
If .Show = -1 Then ' if OK is pressed
selectedFile = .SelectedItems(1) ' get the path of the file
Else
MsgBox "No file selected. Exiting sub."
Exit Sub
End If
End With
' Open the text file with names
Set fso = CreateObject("Scripting.FileSystemObject")
Set ts = fso.OpenTextFile(selectedFile, ForReading)
' Process each line in the text file
Do While Not ts.AtEndOfStream
' Identify the template slide (change 1 to your template slide number)
Set slideTemplate = ActivePresentation.Slides(1)
textLine = ts.ReadLine
slideTemplate.Duplicate
Set newSlide = ActivePresentation.Slides(2)
newSlide.MoveTo toPos:=ActivePresentation.Slides.Count
newSlide.Shapes(shapeName).TextFrame.TextRange.Text = textLine
Loop
'Set slideTemplate = ActivePresentation.Slides(1)
'slideTemplate.SlideShowTransition.Hidden = msoTrue
ts.Close
End Sub
(Optional) Use ChatGPT to Generate the VBA Code
If you don’t have experience working with VBA and don’t want to use the code provided here, you may ask ChatGPT (or any other LLM) to generate the VBA code for you (Pro Tip!). With some prompt engineering, we will have our VBA code ready to be used. Here is a prompt example that you can use as an inspiration:
Prompt #1:
I have created a certificate template in PowerPoint. I want to create a VBA code and Macro that could help me to generate certificates in bulk and populate them from names contained in a text file (names.txt).
The names will be listed one per line in the names.txt file. Here is an example:
John Doe
Jane Doe
Jessica Doe
James Doe
Johnny Doe
Jim Doe
Help to generate the VBA code to process the names.txt file and generate a new slide populating the PowerPoint shape named FullName with each of the names in the text file. A new slide will be created for each line in the names.txt file.
Explain how to set up the Macro that will execute this code.
ChatGPT will suggest you a code. Instead of loading the txt file from a pre-defined path, we decided it would be better to use a File Dialog that let us choose a .txt file from our computer. This way, we may have different .txt files for different events. To support it, you may ask something like this:
Prompt #2:
Tweak the VBA code to add the ability to pick a .txt file from the local computer, by providing a File Dialog box to choose that file.
Now, ChatGPT updated the code by providing a new File Dialog and let the user choose the .txt file when running the Macro.
Here is a preview how your code will look once inserted into VBA.
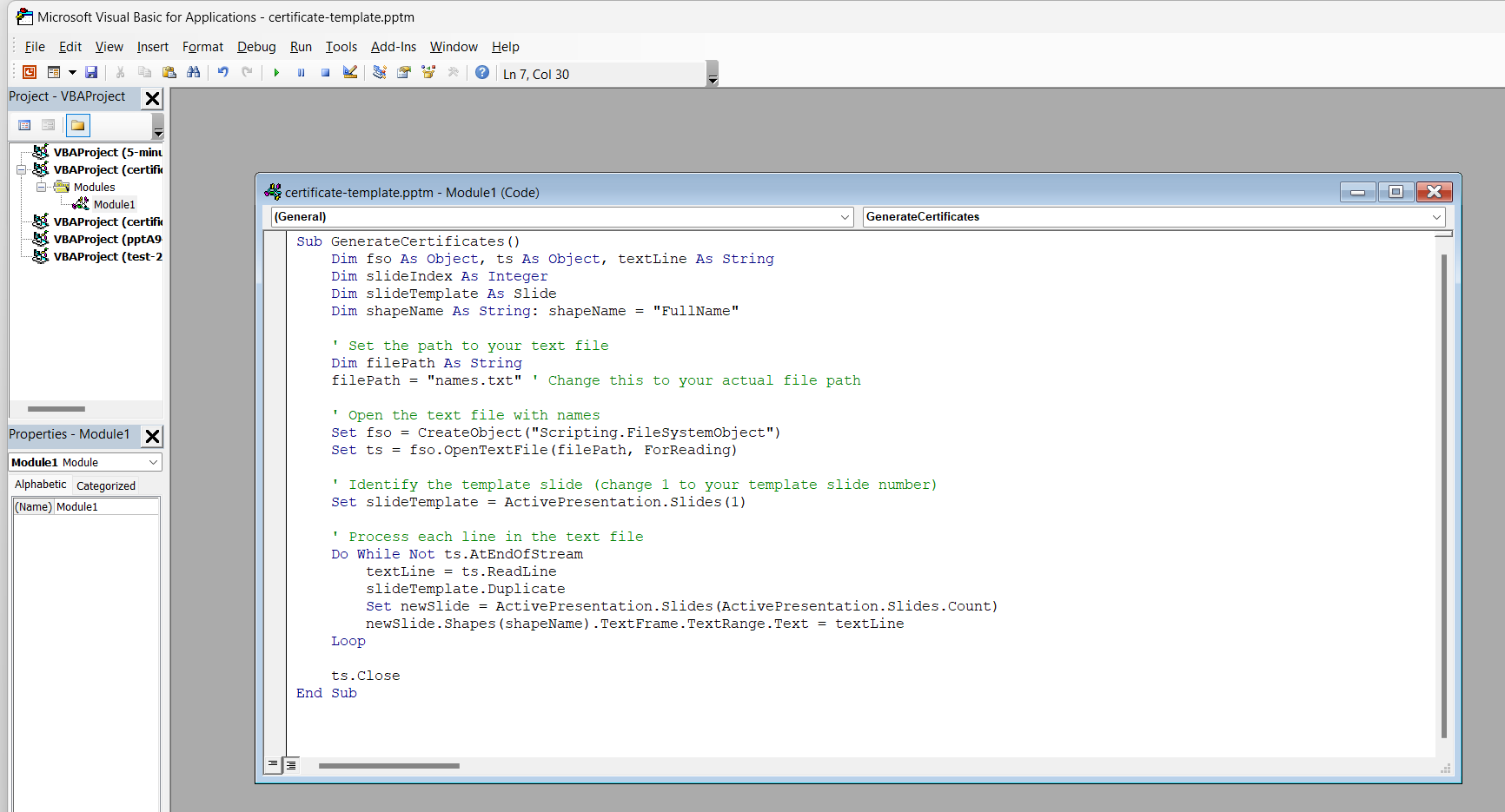
Important: The code uses Scripting.FileSystemObject to open the file so that it will require a reference.
To add this reference, Go to Tools > References and check Microsoft Scripting Runtime option. Otherwise, if you don’t pick this option, you’ll receive an runtime error 5 (Invalid procedure call or argument) when you run the Macro.
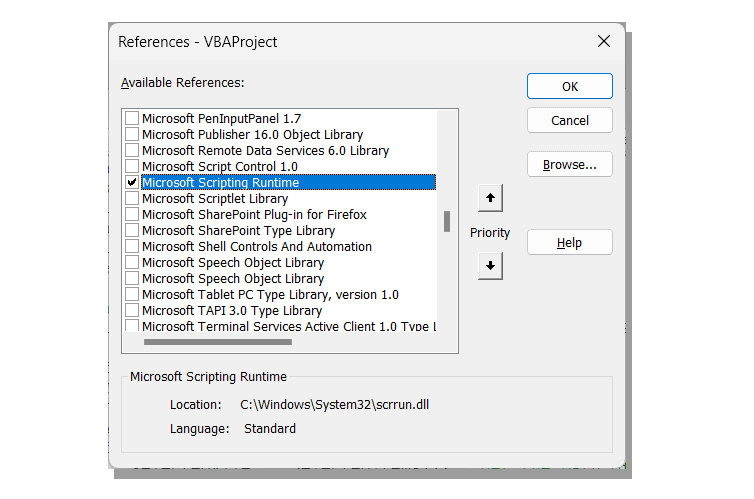
3. Preparing the Data
Next, it’s time to prepare a text file with the list of names that will be used to generate the presentation with the multiple certificate slides. This can be the list of the participant’s name in a webinar, training session, exams, lectures, etc.
Create a new file names.txt and insert the following lines:
John Doe
Jane Doe
Jessica Doe
James Doe
Johnny Doe
Jim Doe
4. Running the Macro Presentation to Generate Multiple Certificates
We are ready to run the macro, pick the file with names, and generate the certificates in bulk.
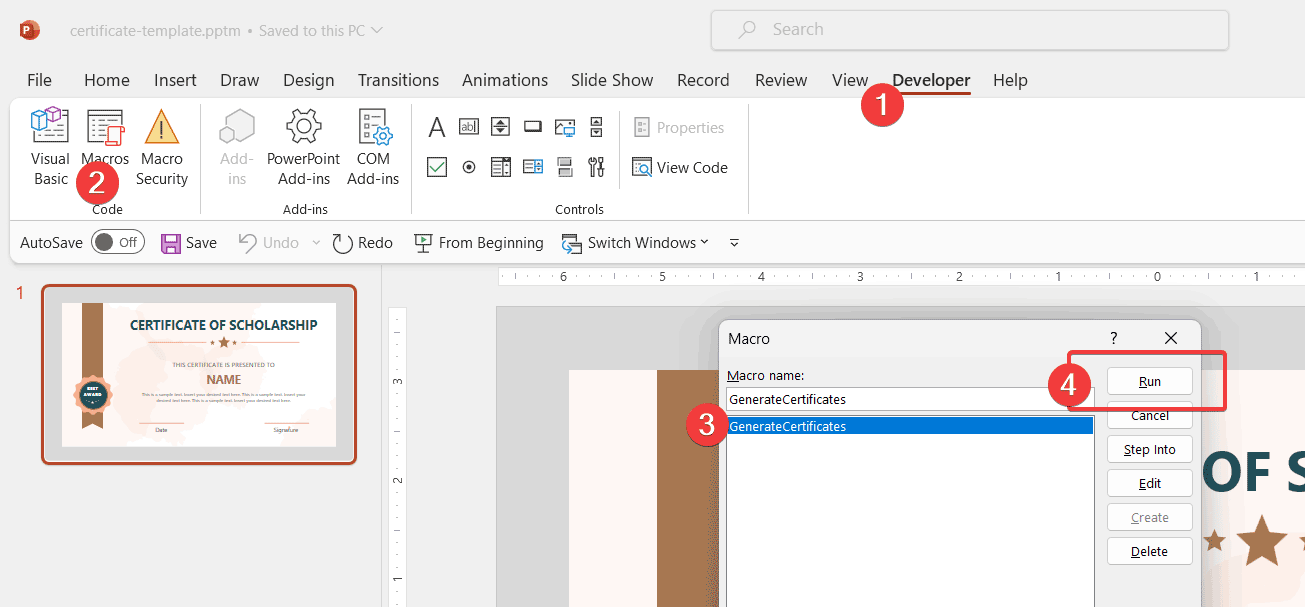
Here is an example showing how the additional slides with the custom names are being generated:
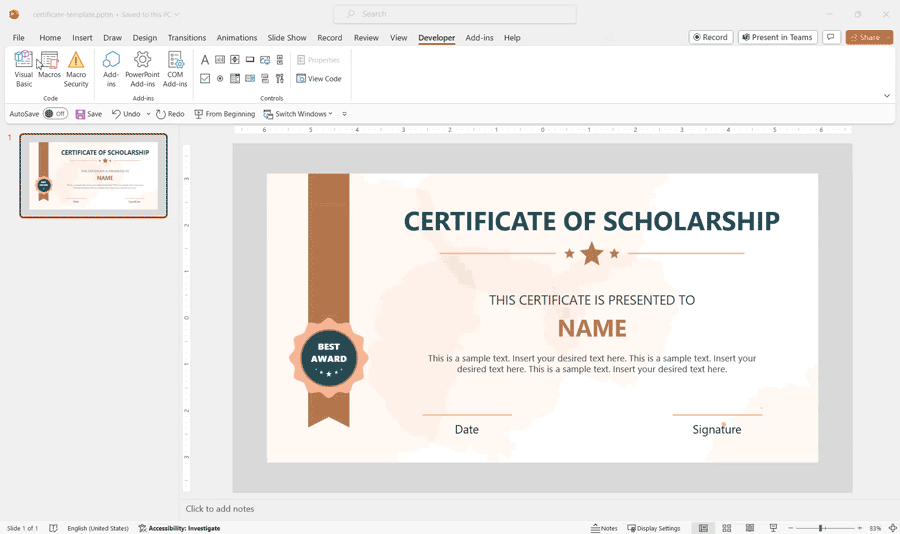
And that’s all!
Now, we have multiple certificate slides generated in PowerPoint (in bulk) using VBA, and from a list of custom names. In our case, the .txt file contained six names; hence, six new slides were added to the end of the presentation. We can now save these certificates as images (to create digital certificates), send for print, or do whatever we need with them.
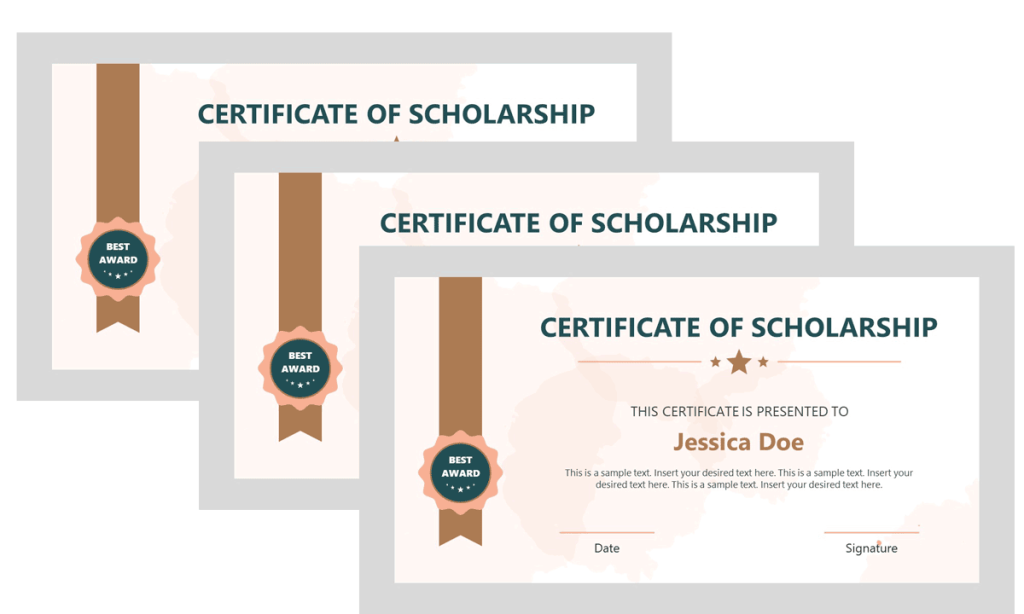
Applications and Use Cases
Whether in educational settings, corporate environments, or public workshops, the ability to quickly produce custom certificates can save a lot of hours of manual work and ensure consistency. Here are several practical applications and use cases where generating certificates in bulk can significantly enhance your productivity.
- Educational Institutions: Automatically generate certificates for students completing courses or workshops. You can also use custom certificate templates for online courses.
- Corporate Training: Quickly produce professional certificates for employees who finish training programs, training sessions, or those who finish the new hire onboarding process.
- Workshops and Conferences: Offer participants instant proof of participation and completion.
- Virtual Seminars: Create digital certificates for participants of virtual seminars and webinar presentations.
Conclusion
Automating certificate generation with VBA in PowerPoint can significantly enhance productivity, reduce errors, and allow organizers to focus on more critical aspects of their events. While you can use PowerPoint to design professional certificatesfrom scratch or by using a certificate presentation template, automating certificate generation can save hours of manual work, especially when you have a large list of participants in your event.